Technology
How to Create Image Segmentation with SVM: Step-by-Step Guide
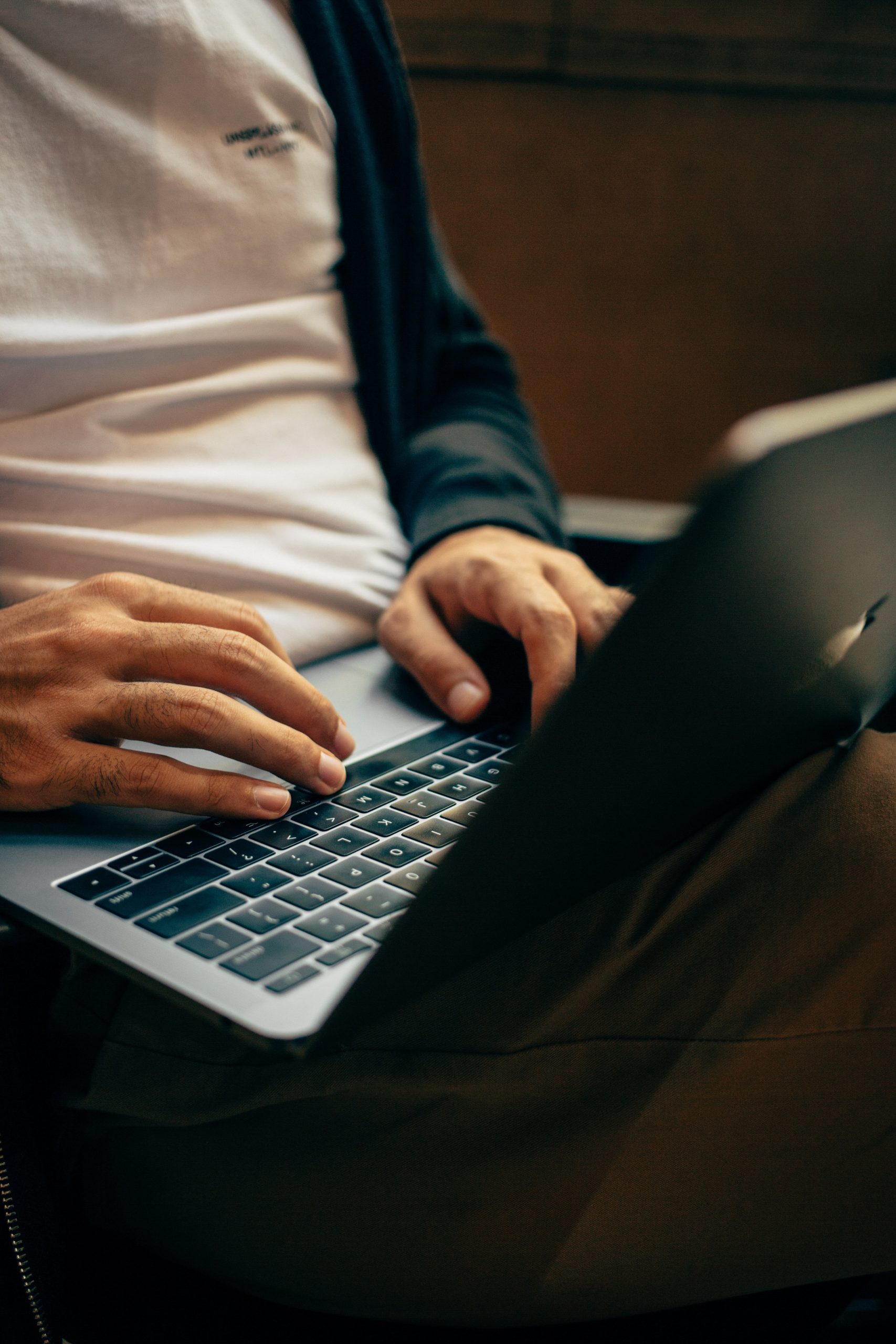
Image segmentation is a fundamental task in computer vision that involves dividing an image into meaningful regions, such as separating objects from their backgrounds. Support Vector Machines (SVM), a powerful machine learning algorithm, can effectively perform image segmentation due to their robustness in handling complex datasets. This guide will walk you through creating image segmentation with SVM, explaining each step and its significance.
What is Image Segmentation?
Image segmentation refers to the process of partitioning an image into different segments or regions based on specific characteristics such as color, intensity, or texture. The primary goal is to simplify the representation of an image and make it more meaningful and easier to analyze.
Why Use SVM for Image Segmentation?
Support Vector Machines are widely used for classification tasks, making them ideal for segmenting images into multiple classes. SVMs are particularly effective for:
Handling high-dimensional data: Image data often involves large feature sets, which SVMs handle well.
Non-linear classification: Using kernel functions, SVM can create non-linear boundaries, allowing complex segmentations.
Good generalization: SVMs minimize classification errors and perform well on unseen data.
Step-by-Step Guide to Image Segmentation with SVM
1. Preprocessing the Image
Preprocessing is crucial to prepare the image for segmentation.
Convert to Grayscale: Simplifies the image data and reduces computational complexity. import cv2
image = cv2.imread(‘image.jpg’)
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Normalization: Standardizes pixel values to improve model performance. normalized_image = gray_image / 255.0
2. Extract Image Features
Features are the input data for the SVM model. Common features include color, texture, and intensity.
Create a Feature Matrix: For grayscale images, pixel intensity serves as a simple feature. For more complex images, consider using additional features like edges or texture descriptors. import numpy as np
height, width = normalized_image.shape
feature_matrix = normalized_image.reshape((height * width, 1))
3. Prepare Training Data
Image segmentation often requires labeled data to train the SVM model. If labels are not available, consider creating them manually or using semi-supervised methods.
Label Assignment: Create labels corresponding to the regions you want to segment. labels = np.zeros((height * width)) # Replace with actual labels
4. Train the SVM Model
Train the SVM using your feature matrix and labels.
Import SVM from Scikit-Learn: from sklearn import svm
classifier = svm.SVC(kernel=’rbf’, C=1.0) # Radial Basis Function (RBF) kernel for non-linear classification
classifier.fit(feature_matrix, labels)
5. Apply the Model to Segment the Image
After training, use the SVM to classify each pixel in the image.
Predict Segmentation: segmented_labels = classifier.predict(feature_matrix)
segmented_image = segmented_labels.reshape((height, width))
6. Visualize the Segmented Image
To understand the segmentation results, visualize the segmented image.
import matplotlib.pyplot as plt
plt.imshow(segmented_image, cmap=’gray’)
plt.title(‘Segmented Image’)
plt.show()
Tips for Improving Segmentation Results
Use Multiple Features: Combining color, texture, and edge information often improves segmentation accuracy.
Optimize SVM Parameters: Adjust hyperparameters like the kernel type (linear, RBF, polynomial) and regularization parameter (C) using grid search.
Preprocessing Techniques: Enhance the image with noise reduction (Gaussian blur) or edge detection (Canny) before feature extraction.
Common Challenges and Solutions
Class Imbalance: If one region significantly outnumbers others, SVM might favor the dominant class. Use class weights to balance training.
Overfitting: Ensure the model generalizes well by using cross-validation and tuning hyperparameters.
Complex Boundaries: For intricate segmentations, experiment with different kernel functions to capture non-linear boundaries effectively.
Creating image segmentation with SVM involves several well-defined steps, from preprocessing and feature extraction to training the model and applying it to segment images. SVM’s strength in handling high-dimensional data and non-linear boundaries makes it a powerful tool for this task. By following this guide and applying best practices, you can achieve accurate and meaningful image segmentation, unlocking valuable insights for various applications like medical imaging, object recognition, and more.
